# 8. NgForm
Since you imported the NgForm directive into the root module, Angular automatically puts the directive on all the form tags.
NgForm holds the data about the values, validation states, and classes of the form.
You can see the change in the state of a form field by attaching a local reference. For example:
<input type="text" name="name" [(ngModel)]="carModel.name" required #trackName>
{{trackName.className}}
#trackName allows us to track the classes of the field:
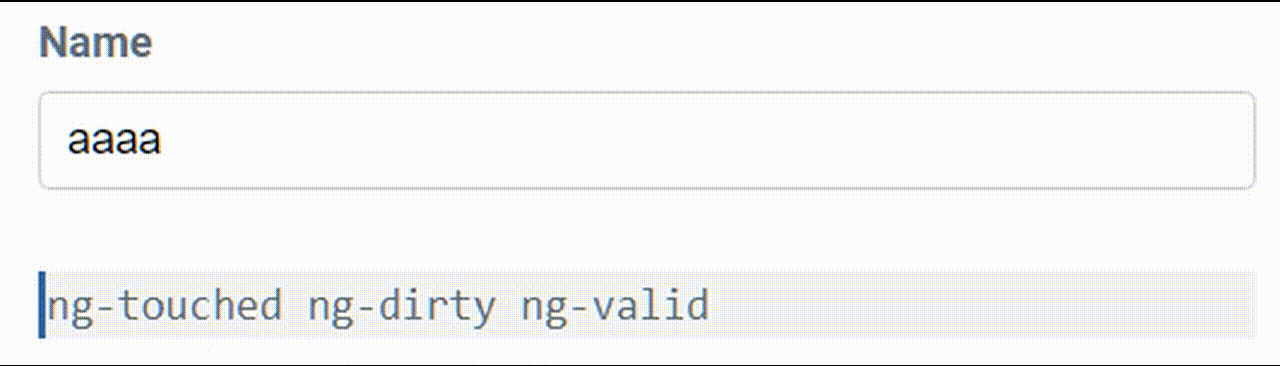
Now you can see the classes that Angular attaches to the fields.
Before touching the form:
- ng-untouched since you haven't touched the field
- ng-pristine since you haven't changed the value
- ng-invalid because the field is required but it still doesn't have a value
Enter the field, then leave it without changing its value, and you will get the following combination of classes:
- ng-touched because you entered the field
- ng-pristine since you haven't changed the value
- ng-invalid because the field is required but it still doesn't have a value
Start typing inside the field to see the following classes:
- ng-touched because you entered the field
- ng-dirty since you changed the field's value
- ng-valid because the field passes the validation rule
As you can track the status of the form fields using local reference, you can follow the entire form in the same way.
Attach a local reference to the opening tag of the field:
<form #carModelForm="ngForm">
Add the following code beneath the form to track the state and values in real-time:
{{carModelForm.value | json}}
to see the values of the fields change as you type.
To track the validity of the form.
{{carModelForm.valid}}

All the form fields are required, and so you need to enter values to all the fields for the validation state to change from false to true.